JavaScript API Additional Information / HTML5 Content Tips and Tricks
Supported Platforms:
Pitcher Impact iOS
Pitcher Impact Windows
Pitcher Impact Android
Not Supported Platforms:
Pitcher Connect
This article is currently being developed. We will expand on how to develop custom HTML5 content which is not based on automatically converted standard file formats.
Close function
Usually for closing presentations we use "Ti.App.fireEvent('closeScrollWeb');". As we are working in a custom HTML5 piece this will not work. We need to use "Ti.App.fireEvent('closeOpenModal', {});" see screen below:
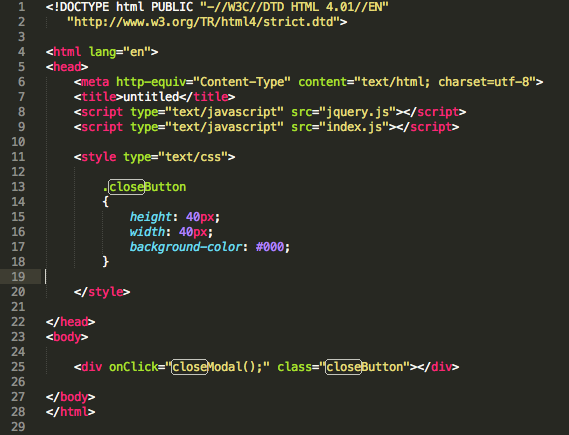
Pitcher XLS Translation Feature for Interactive HTML5 Content
Follow the Injectin Sample that is attached below with respect to the following points:
Translations have to be in CSV not JSON
lang folder CSV file names have to match (see screenshot)
initial CSV has to be UTF-8
For multi language presentations please check: http://i18njs.com
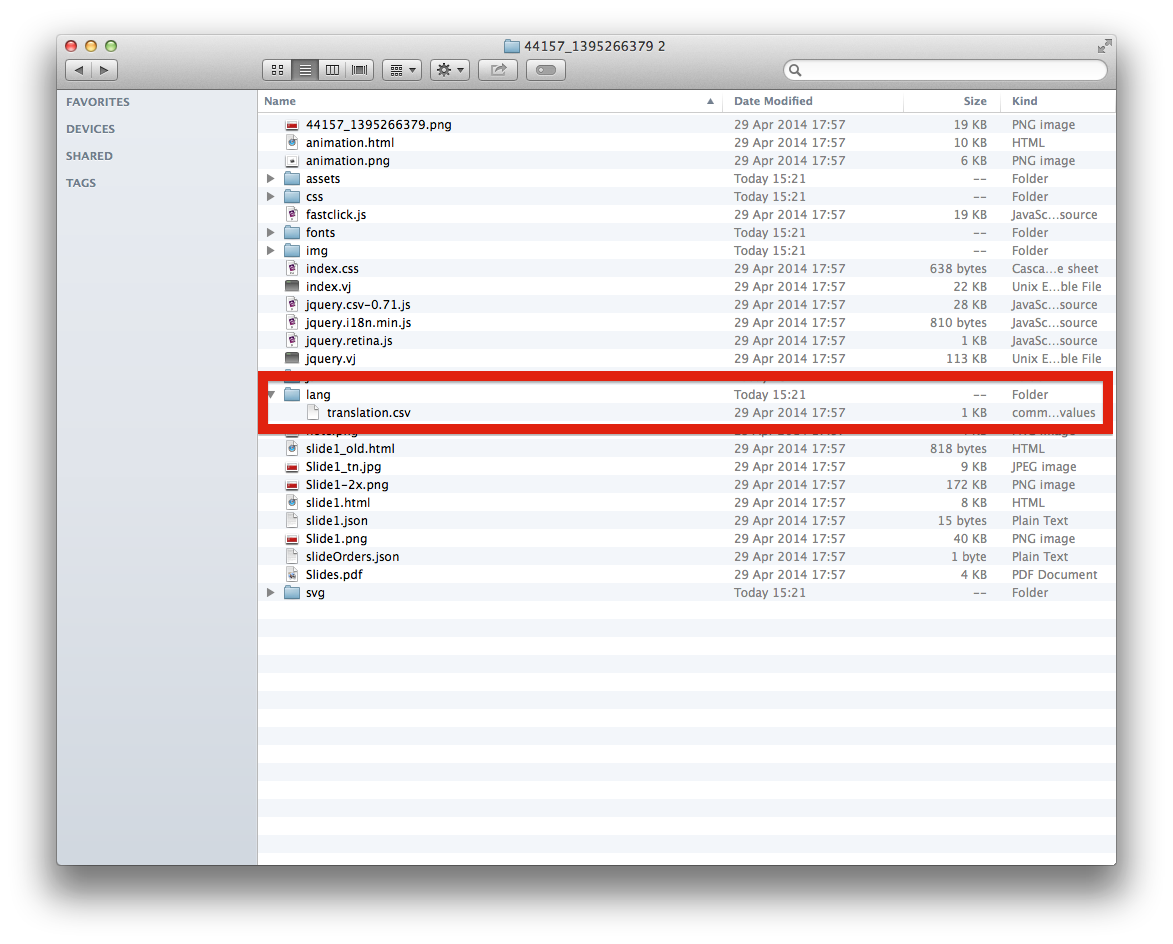
Send Email with pre-defined attachment and body text
function send_email(subject, message, attachment){
Ti.App.fireEvent('sendEmail', {
'subject': subject,'messageBody': message,
'isHTML':true,
"toReceipients":["info@pitcher.com","support@pitcher.com"],
'fileURLs':attachments }); }
As you can see, you may also predefine the recipients, as well as attach a file to this email.
For attaching files, the best usecase scenario is to generally genarating a pdf on the iPad, which may be a sales form result or a filled out questionaire. I will provide additional information for generating pdf files and attaching to emails just in case if you might need it in the future. For this example we prepare a html layout like a web page, we define this html code in a variable and call the function named generatePDF(htmlString); after the pdf creation is completed successfully, Pitcher automatically calls the function named gotPDF, in which step we call the send email function with this file as an attachment.
Note: If you want to include CSS rules and images in the pdf template, please include them as inline style rules and inline base64 images to be rendered properly. External resources can not be loaded during PDF generation process.
function generatePDF(htmlString){
if(htmlString!=null){
Ti.App.fireEvent("generatePDFfromString",
{"htmlString":htmlString,"fileName":"SalesForm.pdf"}); } };
function gotPDF(filePath){ Ti.App.fireEvent("sendEmail",{
"fileURL":filePath,"subject":"Pitcher - Sales Form",
"messageBody":"Message Body",
"toReceipients":["info@pitcher.com","support@pitcher.com"] });};
Delaying image - HTML switch.
As explained earlier, Pitcher uses PNG files in the folder to show while HTML file is being loaded. On DOM Ready event (or body onload) these images are hidden and HTML is shown. In certain cases (like where page is built using AngularJS) even though dom ready event is fired, page might not be ready.
This might cause flickers.
To prevent this, you need to
First tell Pitcher to ignore DOM ready event
Then when page is ready tell Pitcher to do the PNG -> HTML switch.
To tell Pitcher to ignore DOM ready event:
Please add following function to your JS library
function shouldHoldOffOnImageSwap(){ return true;}
Pithcer by default calls this function, if this function does not exist, returns null, or returns false Pitcher uses DOM Ready event.
To Tell Pitcher to Swap Image and HTML When Page is Ready:
Please fire the following event when page is 100% ready
Ti.App.fireEvent('loadFinished');
iPhone Remote Control - Presentation Interaction
To dispatch touch events from remote on the custom HTML5 content a touch synthesizer function needs to be added on the content.
Find one example function below:
function touchSynth(x,y,mode){
if(x<1 && y<1){
x = x*$(window).width();
y = y*$(window).height(); }
var targetElement = document.elementFromPoint(x, y);
var modeString = '';
switch(mode){
case 'tb':
modeString = 'touchstart';
break;
case 'tm':
modeString = 'touchmove';
break;
case 'te':
modeString = 'touchend';
break; }
var identifier = new Date().getTime();
var touch = document.createTouch(window,
targetElement, identifier, x, y, x, y);
var touches = document.createTouchList(touch);
var targetTouches = document.createTouchList(touch);
var changedTouches = document.createTouchList(touch);
var e = document.createEvent('TouchEvent');
e.initTouchEvent(modeString, true, true, window, 1,
x, y,//screen x, y, false,false, false, false,
touches, targetTouches, changedTouches,1, 0);
//var simulatedEvent = document.createEvent("TouchEvent");
targetElement.dispatchEvent(e);
if(modeString == "touchend"){
var simulatedEvent = document.createEvent("MouseEvent");
simulatedEvent.initMouseEvent("click", true, true, window, 1,
x, y,//screen x, y, false,
false, false, false, 0/*left*/, null);
targetElement.dispatchEvent(simulatedEvent); }
}
Free text search
Pitcher conversion engine extracts text from Powerpoint or Keynote files during conversion. If you're using custom HTML5 development, slide tags need to be added manually so free text search engine can match with the slides. In slide{x}.json please add the child array object searchKeywords with the keywords
e.g.
{ "hotspots": [], "slideName": "Slide Name 1",
"searchKeywords": [ "Keyword 1",
"Keyword 2",
"Keyword 3" ]}
Disabling Pitcher double click function on presentation
you can add the following code to your script library to tell Pitcher to ignore double clicks on elemens on the page, such as calculator entry points or links
function preventDoubleTap(x,y){ ....
return "true"; }
Caching behaviour of Impact
By default Pitcher Impact caches like a dice (2 next slides, 1 previous slide on both dimensions), totaling up to 7 slides in the memory including the current slide.
this can cause some memory issues on certain devices and certain content. To limit this number, you can use maxCache parameter in setup.json. see example below:
{ "hasReference": false,
"hideHotspots": true, "maxCache": 1,
"maxCharacter": "15", "menuOneButtonColor": "#3B93CB",
"menuOneColor": "#DDDDDD", "menuOneHeight": "44",
"menuOneItems": null, "menuTwoButtonColor": "#3B93CB",
"menuTwoColor": "#DDDDDD", "menuTwoItems": null,
"navMode": 0, "scrollBar": false,
"showMenuOnTop": false,
"showNavigation": false,
"widthPerItem": "250"}
Getting file information from Pitcher file system.
Getting All Files in the Pitcher File System
Supported Platforms
Pitcher Impact iOS
Pitcher Connect
Pitcher Impact Windows
Pitcher Impact Android
function getAllFiles(){
Ti.App.fireEvent("getPitcherFiles",
{"callBackFunc": "console.log", "fullData": true});}
Searching a file in the Pitcher File System from file keywords
Supported Platforms
Pitcher Impact iOS
Pitcher Impact Windows
Pitcher Impact Android
Unsupported Platforms
Pitcher Connect
function searchFile(searchKeywords){ Ti.App.fireEvent("searchPitcherFile",
{"callBackFunc":"console.log","keywords":searchKeywords,"rangeCheck":true});}
Getting detailed information about a specific file with a file ID
Supported Platforms
Pitcher Impact iOS
Pitcher Impact Windows
Pitcher Impact Android
Unsupported Platforms
Pitcher Connect
function getFileDetails(fileID){
Ti.App.fireEvent("getPitcherFile",
{"callBackFunc":"console.log","fileID":fileID});}
Getting current geo location coordinates of the user, will return zero "0" values if the user didn't give permission for geo location.
Getting detailed information about a specific file with a file ID
Supported Platforms
Pitcher Impact iOS
Pitcher Impact Windows
Pitcher Impact Android
Unsupported Platforms
Pitcher Connect
/* If the device is set to share its
/*geo location, this data can be accesses from the interactive document */
function getGeoCordinates(){
Ti.App.fireEvent('getCoordinates',{'callBack':'console.log','source':'modal'});}
Force a Salesforce.com resync after sending values via createSFDC and updateSFDC functions.
Supported Platforms
Pitcher Impact iOS
Pitcher Impact Windows
Pitcher Impact Android
Unsupported Platforms
Pitcher Connect
function syncCRM(){ Ti.App.fireEvent("resyncData");}
Sample getInfo
function parameters for Presentation type interactive documents;
This are the sample parameters pushed as params the user is not logged into SFDC.
{ "deviceName": "Pitcher Test Device",
"categoryLogo": null, "langV": "EN", "metadata": "PitcherDebug, PitcherAgency"}
This are the sample parameters pushed as params while logged into SFDC but not in a meeting session.
{ "deviceName": "Pitcher Test Device",
"categoryLogo": null, "salesForceUser": {
"attributes": { "type": "User",
"url": "/services/data/v29.0/sobjects/User/005i0004336iTnIAAU" },
"Name": "PoC SSO User", "Email": "pitcher-poc@pitcher.com",
"UserRoleId": null, "Manager": null, "ProfileId": "00ei0001801ZQA3AAO",
"LanguageLocaleKey": "en_US", "Id": "005i0004336iTnIAAU" },
"langV": "EN", "metadata": "PitcherDebug, PitcherAgency"}
This are the sample parameters pushed as params while logged into SFDC and during a meeting session.
{
"deviceName": "Pitcher Test Device",
"categoryLogo": null,
"salesForceUser": {
"attributes": {
"type": "User",
"url": "/services/data/v29.0/sobjects/User/005i0004336iTnIAAU" },
"Name": "PoC SSO User",
"Email": "pitcher-poc@pitcher.com",
"UserRoleId": null,
"Manager": null,
"ProfileId": "00ei0001801ZQA3AAO",
"LanguageLocaleKey": "en_US",
"Id": "005i0004336iTnIAAU" },
"langV": "EN",
"metadata": "PitcherDebug, PitcherAgency",
"accountName": "Health Clinic", "accountObject": {
"attributes": { "type": "Account",
"url": "/services/data/v29.0/sobjects/Account/001i000001UOOxpAAH" },
"Id": "001i000001UOOxpAAH", "Name": "GenePoint",
"Type": "Customer - Channel", "BillingCity": "Mountain View",
"Phone": "(650) 867-3450", "BillingState": "CA",
"BillingStreet": "345 Shoreline Park Mountain View, CA 94043 USA",
"BillingCountry": null, "ShippingCity": null,
"ShippingState": null,
"ShippingStreet": "345 Shoreline Park Mountain View, CA 94043 USA",
"BillingLatitude": 37.428434,
"BillingLongitude": -122.0723816,
"Lat__c": 37.428434, "Long__c": -122.0723816 },
"contactName": "Edna Frank", "contactObjects": [ {
"attributes": { "type": "Contact",
"url": "/services/data/v29.0/sobjects/Contact/003i000002WbZFSAA3" },
"Id": "003i000002WbZFSAA3", "FirstName": "Edna",
"LastName": "Frank", "Name": "Edna Frank", "Account": {
"attributes": { "type": "Account",
"url": "/services/data/v29.0/sobjects/Account/001i000001UOOxpAAH" },
"Id": "001i000001UOOxpAAH" },
"Email": "efrank@genepoint.com", "Segment__c": "A1",
"segment": "A1" } ]}
Sample getParameters function parameters for Zip type interactive documents;
This are the sample parameters pushed as params the user is not logged into SFDC.
{ "account": null, "user": null, "langV": "EN", "currentContactObjects": []}
This are the sample parameters pushed as params while logged into SFDC but not in a meeting session.
{ "account": null,
"user": { "attributes":
{ "type": "User",
"url": "/services/data/v29.0/sobjects/User/005i0004336iTnIAAU" },
"Name": "PoC SSO User", "Email": "pitcher-poc@pitcher.com",
"UserRoleId": null, "Manager": null,
"ProfileId": "00ei0001801ZQA3AAO",
"LanguageLocaleKey": "en_US", "Id": "005i0004336iTnIAAU" },
"langV": "EN", "currentContactObjects": []}
This are the sample parameters pushed as params while logged into SFDC and during a meeting session.
{ "account":
{ "attributes":
{ "type": "Account",
"url": "/services/data/v29.0/sobjects/Account/001i000001UOOxpAAH" },
"Id": "001i000001UOOxpAAH",
"Name": "GenePoint",
"Type": "Customer - Channel",
"BillingCity": "Mountain View",
"Phone": "(650) 867-3450",
"BillingState": "CA",
"BillingStreet": "345 Shoreline Park Mountain View, CA 94043 USA",
"BillingCountry": null,
"ShippingCity": null,
"ShippingState": null,
"ShippingStreet": "345 Shoreline Park Mountain View, CA 94043 USA",
"BillingLatitude": 37.428434,
"BillingLongitude": -122.0723816,
"Lat__c": 37.428434, "Long__c": -122.0723816 },
"contact": { "attributes": {
"type": "Contact",
"url": "/services/data/v29.0/sobjects/Contact/003i000002WbZFSAA3" },
"Id": "003i000002WbZFSAA3",
"FirstName": "Edna", "LastName": "Frank",
"Name": "Edna Frank", "Account": {
"attributes": { "type": "Account",
"url": "/services/data/v29.0/sobjects/Account/001i000001UOOxpAAH" },
"Id": "001i000001UOOxpAAH" },
"Email": "efrank@genepoint.com",
"Segment__c": "A1", "segment": "A1" },
"user":
{ "attributes":
{ "type": "User",
"url": "/services/data/v29.0/sobjects/User/005i0004336iTnIAAU" },
"Name": "PoC SSO User", "Email": "pitcher-poc@pitcher.com",
"UserRoleId": null, "Manager": null,
"ProfileId": "00ei0001801ZQA3AAO",
"LanguageLocaleKey": "en_US", "Id": "005i0004336iTnIAAU" },
"langV": "EN", "currentContactObjects": [
{ "attributes": {
"type": "Contact",
"url": "/services/data/v29.0/sobjects/Contact/003i000002WbZFSAA3" },
"Id": "003i000002WbZFSAA3", "FirstName": "Edna",
"LastName": "Frank", "Name": "Edna Frank",
"Account": { "attributes": {
"type": "Account",
"url": "/services/data/v29.0/sobjects/Account/001i000001UOOxpAAH" },
"Id": "001i000001UOOxpAAH" },
"Email": "efrank@genepoint.com",
"Segment__c": "A1", "segment": "A1" } ]}
WKWebView vs. UIWebView
Pitcher uses UIWebView as a default engine until end of 2020 for backwards compatibility and legacy support. To switch to WKWebView simply add useWK as a keyword to your interactives.